Add watermark to pdf and compress in Python 3 using PyPDF2
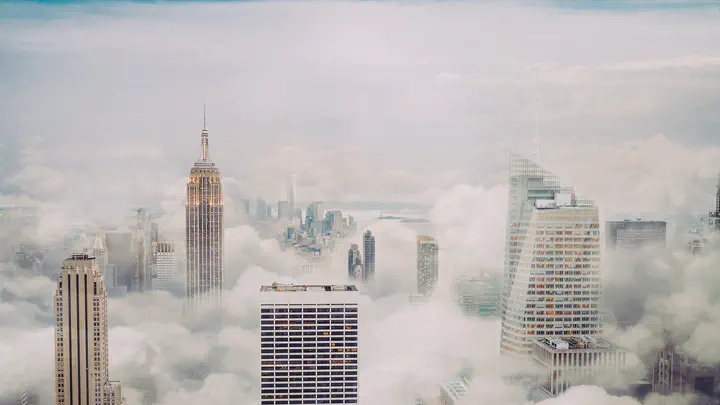
In this post, I will share the Python 3 version of PyPDF2
to add watermark to pdf and compress the pdf file. On the internet, you can find many similar examples of adding watermark to pdf using PyPDF2
but most of them are for Python 2.7. I have updated the code to work with Python 3.
The popular way is to merge two files. I assume you have a watermark pdf file with same page size of the input file.
The code logic is simple. We will read the input pdf file and watermark pdf file. Then we will merge the watermark page to each page of the input pdf file. Finally, we will write the merged file to a new pdf file. The only thing is to compress the content stream of each page to reduce the file size. The file size before and after compression is very similar. If not compress, the size can be 3x or 4x of the original file.
from PyPDF2 import PdfFileMerger, PdfReader, PdfWriter
pdf_file = "input.pdf"
watermark = "watermark.pdf"
merged = "merged.pdf"
with open(pdf_file, "rb") as input_file, open(watermark, "rb") as watermark_file:
input_pdf = PdfReader(input_file)
watermark_pdf = PdfReader(watermark_file)
watermark_page = watermark_pdf.pages[0]
output = PdfWriter()
for i in range(len(input_pdf.pages)):
pdf_page = input_pdf.pages[i]
pdf_page.merge_page(watermark_page)
pdf_page.compress_content_streams()
output.add_page(pdf_page)
with open(merged, "wb") as merged_file:
output.write(merged_file)